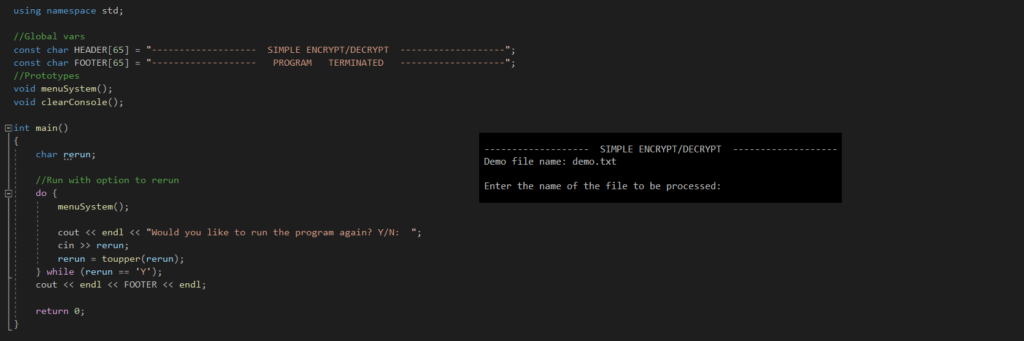
I mentioned this project in my last post, but one of the security tools I enjoyed writing the most was the simple encryption tool I wrote in C++. Toward the end of my last programming course at Granite State College, I learned how to write a simple encryption function in C++. With this project, I sought to stage a user-friendly interface around this encryption function, incorporating a menu and a decryption feature to reverse the encryption.
This project taught me something important about encryption algorithms – they’re not that complicated. Not that they can’t be complicated, but they’re only as complicated as you need them to be. I guess this seems fairly common-sensical, but it’s something that hadn’t really occurred to me. Writing an encryption algorithm always seemed like such a daunting task, but as you can see from the following encryption class code…
class Encrypt : public FProc
{
int eKey;
public:
Encrypt(const string& inName, const string& outName, int key)
: FProc(inName, outName)
{
eKey = key;
cout << "Encryption key successfully applied." << endl;
}
char transform(char ch) const override
{
/* Replace this algorthim to alter
the complexity of the encryption */
return ch + eKey;
}
};
…it can be as simple as that. All this class does is accept a numerical encryption key value and add it to the character value of each character in the designated file. Of course, this simple return ch + eKey; can be replaced by a more complex algorithm (as noted in the comment above), but at its core, this is all an encryption algorithm needs to be. And the cool thing about encryption is that it just needs to be reversed in order to decrypt the file. Another commonsense statement from yours truly, I know, but I think its so cool that you can just implement the following line of code in order to decrypt this file:
return ch - eKey;
In fact, my decryption class is exactly the same as my encryption class, with the only difference being the operator, changing from addition to subtraction. This one line is my favorite part of this program, particularly because it isn’t anything special. I’m so intrigued by the idea that encryption can be this simple. Not only that, but I love that the rest of the code in this program works as a framework around this line. This line can be hot swapped with encryption algorithms of varying complexity, and as long as the decryption class accomplishes the opposite, the program will still function as intended.
In the future, I intend to use this program as a foundation for working on more complex encryption algorithms. Writing this program was a great way for me to hone my C++ skills and learn how basic encryption works. At the time of this post, this program is a little over a month old as it was posted June 20, 2022. Looking back, I’m still pretty happy with the structure of the code, the comments, and the function of the program. The only think I would like to do in the future is implement a basic GUI for this program. Developing graphical user interfaces is a part of programming that I have very little experience with (only minor experience with UWP and WPS for C#). Developing a front end, even if I have to rewrite the entire back end thing in a different language, is probably my next step in fine tuning this program.
If you’re interested, please take a look at SimpleEncryption v1 and some of the other code I’ve uploaded to my GitHub. I’m always open to constructive criticism and feedback to help me improve as a programmer and a future security professional.
As always, thanks for stopping by!
-Kyle